Find and fix production issues, before customers notice
- Catch breaking changes and critical errors
- Analyze logs, metrics, traces and request payloads
- Monitor performance and uptime of both APIs and external APIs
Trusted by 5000+ developers at proactive engineering companies
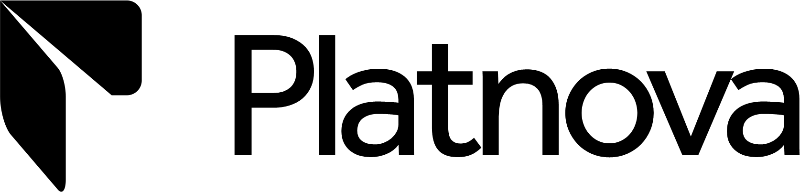
"Easy onboarding and they added an integration just for our use case, thanks again! We didn't have insights into our api load before and this helps very much."

Sebastian Zwach
founder of Neorent GmbH
"We had a major incident, and our tech support could see via APItookit which third-party integration partner was responsible, and could take action without needing the engineering team's help"
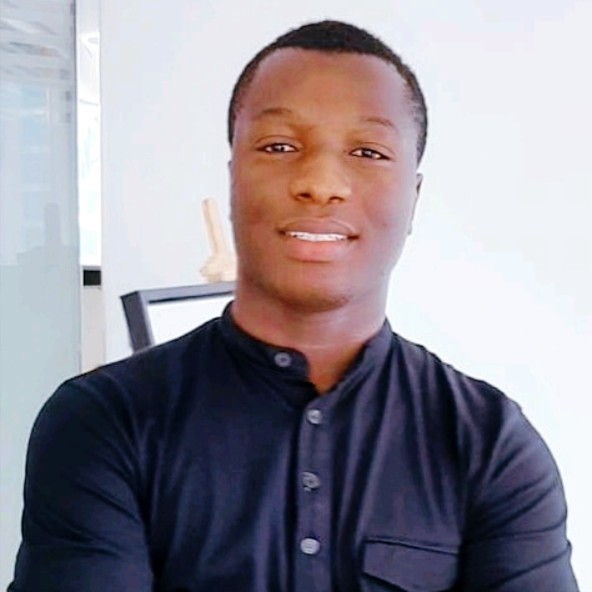
Joshua Chinemezum
CEO of Platnova
APItoolkit offers the best features of Datadog, Sentry, Newrelic, etc., at 1/20 the cost. We train private models on your real-time logs, events, and API request payloads to catch errors and breaking or incremental changes that are impossible to detect on any other platform. Thanks to our unique crytography based algorithms.
You can store all your data in your own S3-compatible storage, giving you complete ownership and letting you query or ask questions of your data forever at no extra storage cost.
Monitoring and Observability,
built to understand your entire stack
Just because you don't see an error, doesn't mean it's not happening. That's why we built both active
and passive monitoring--to keep you informed of the different systems you maintain.
Put AI to work. seriously
Generate queries, create visualizations, fix bugs, monitor logs, payloads, and kickstart whole analyses - all from a prompt.
Learn moreWeekly reports that contain exactly what needs attention
We tell you want errors popped up, which services or endpoints got slower, which weird log appeared, etc.
Query using natural language
Ask questions about your data, your systems or logs without learning some strange query langauge.
Breaking and incremental change detection in APIs (yours or thirdparties)
No need to stare at logs all day. See the errors, anomalies, etc which appeared in the last week.
Built with features that matter to engineers like us
Collect, store, and analyze every single log or event
without limits on a platform that gives you complete control.
Observability & monitoring
API management
Alerts and Monitors
Logs and Traces
Query all your logs and correlate them with trace breakdowns or the requests which triggered the logs.
Learn moreErrors and Performance
Monitor API errors and performance in real time. Detect issues instantly, analyze trends, and optimize response times with detailed insights. Improve reliability with automated tracking, alerting, and in-depth analytics to ensure a seamless user experience.
Learn moreMetrics and dashboards
Define and track custom metrics tailored to your API needs. Gain actionable insights, monitor key performance indicators, and optimize efficiency with real-time data visualization and alerts. Make data-driven decisions to enhance your API’s performance and reliability.
Learn moreAPI Catalog and Docs
Organize, manage, and document your APIs effortlessly with a dynamic API catalog. Provide clear, up-to-date documentation, improve discoverability, and streamline onboarding for developers with interactive guides, code samples, and real-time updates.
Learn moreAnomalies
Detect API changes and anomalies in real time. Stay ahead of unexpected behavior with automated monitoring, instant alerts, and detailed insights. Ensure stability, prevent disruptions, and maintain seamless performance with proactive change detection.
Learn moreMonitors and healthchecks
Ensure API reliability with automated monitors and health checks. Continuously track uptime, detect failures early, and receive instant alerts. Keep your services running smoothly with real-time insights and proactive issue resolution.
Learn moreAlerts and notifications
Stay informed with real-time alerts and comprehensive reports. Customize alert channels and receive detailed analytics to monitor your API's performance and reliability effectively.
Learn moreDaily or weekly reports
Receive comprehensive daily or weekly reports summarizing API performance, errors, and key metrics. Get actionable insights delivered to your inbox to stay ahead of potential issues.
Learn moreFlexible deployment options for every company
Regardless of your company's size or compliance requirements, APItoolkit operates
within your business and regulatory constraints.
780+ Integrations with your favorite tech stacks,
powered by OpenTelemetry
View all integrations.














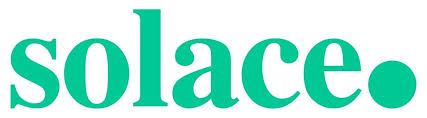
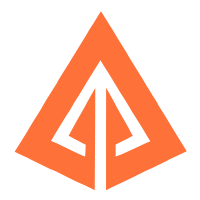
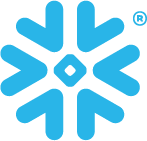
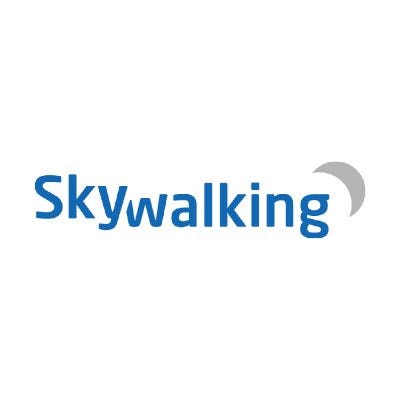
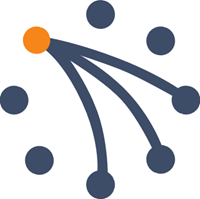
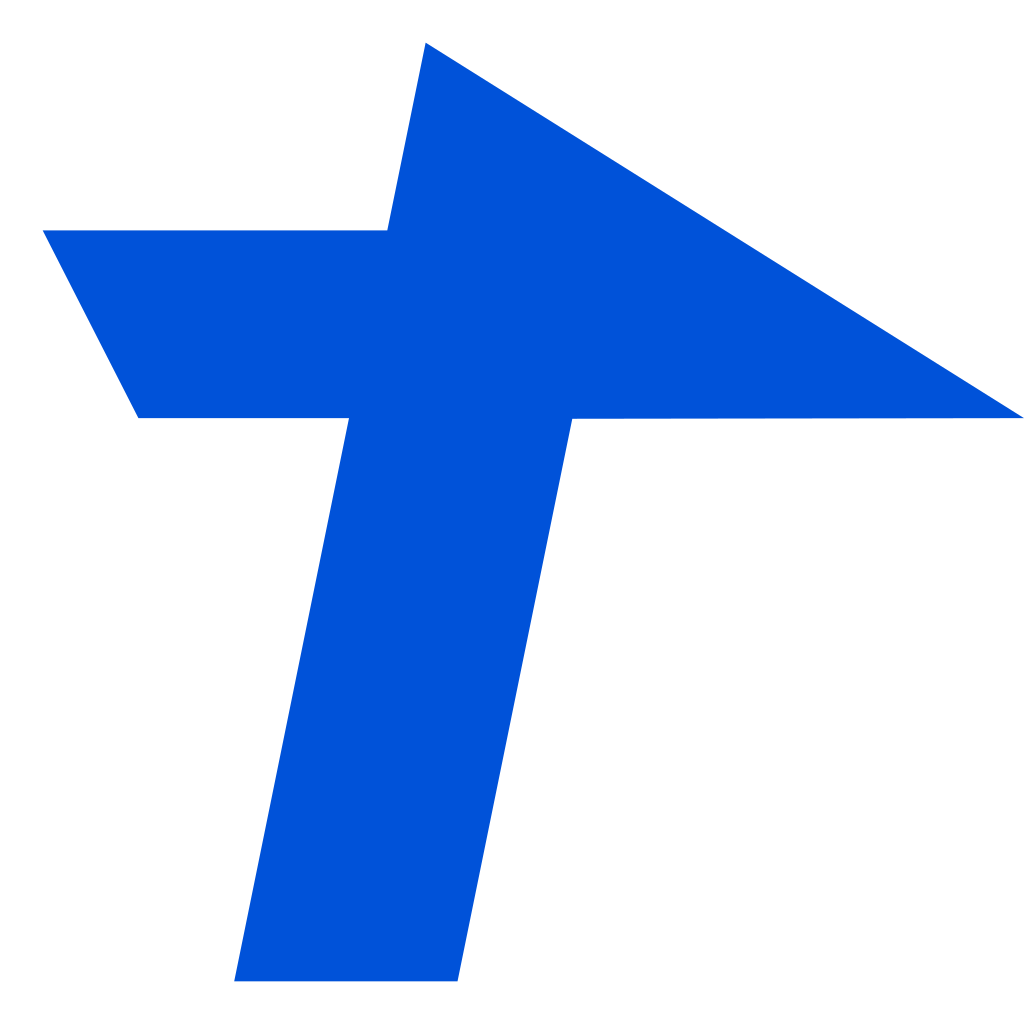
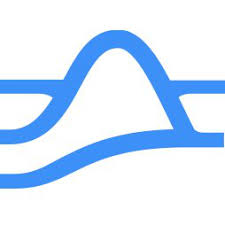
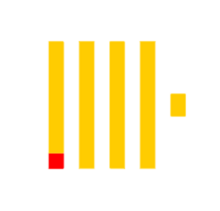
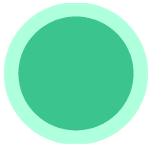

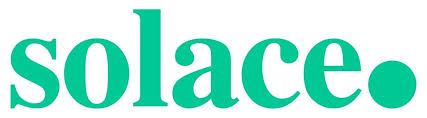
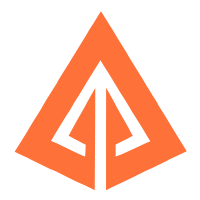
Real results for real companies
View all case studies.We had a major upgrade planned for our iOS/Android app... After adding APItoolkit to our laravel app, we could see every new route and request. It gave me and the dev team complete confidence that our users weren't being affected by our changes.
... became the smoothest and LEAST STRESSFUL rollouts we have ever done. Really an amazing tool and now a permanent part of our workflow. Thank you!!
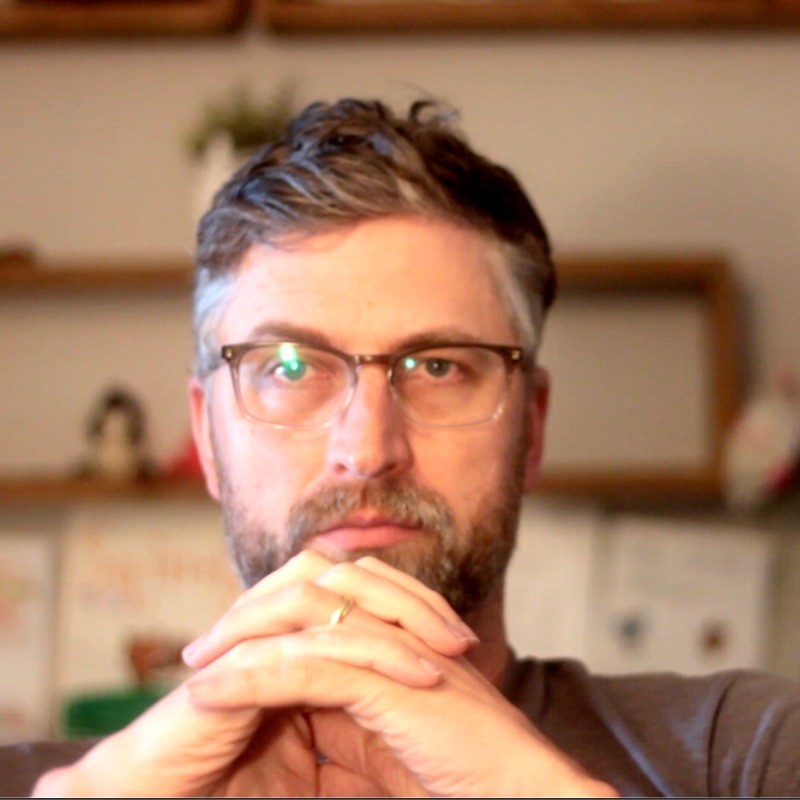
Lazarus Morrison
Founder of Community Fluency
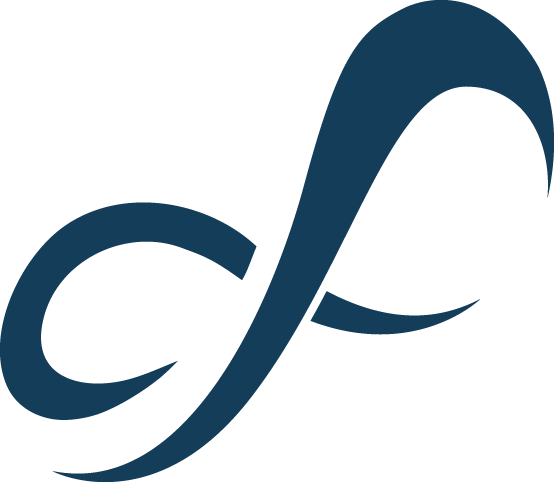
The best observability tool we use today at Woodcore, APItoolkit notifies us about any slight change that happens on the system.
Most especially, for the features we utilise today on APItoolkit, would cost us a lot more elsewhere.
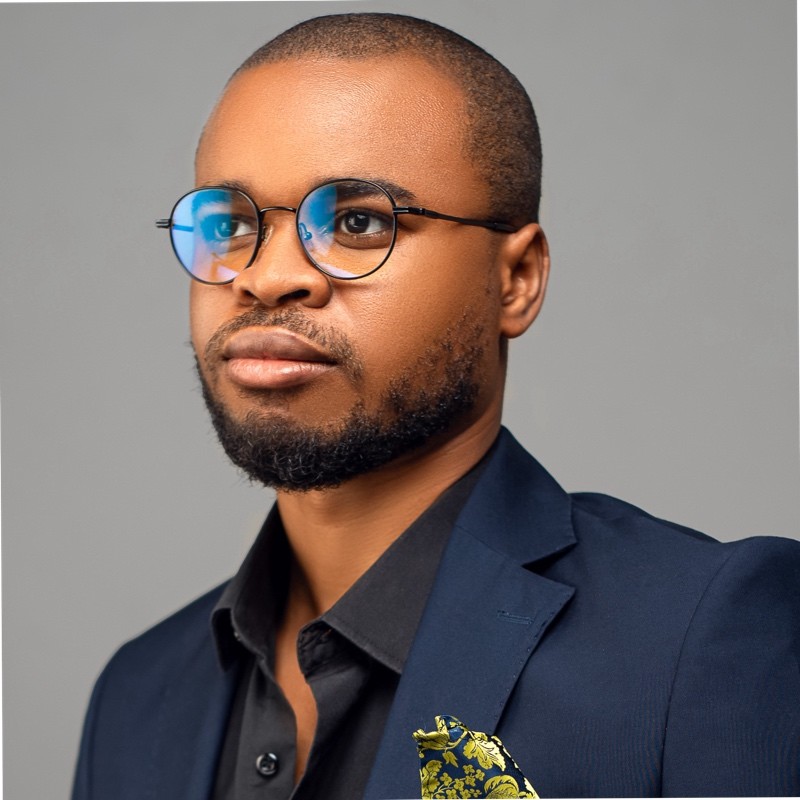
Samuel Joseph
CEO of Woodcore
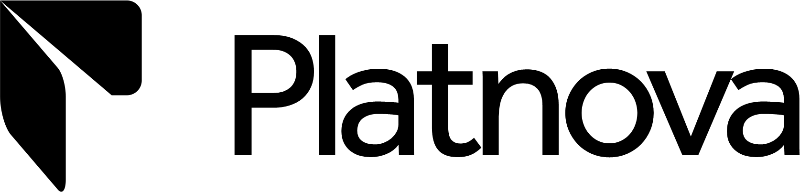
I fell for ApitoolKit because it integrated effortlessly with my application and provided valuable API insights. Whenever I needed help, the team was always ready to listen and resolve any issues. That's why we pay for their service.
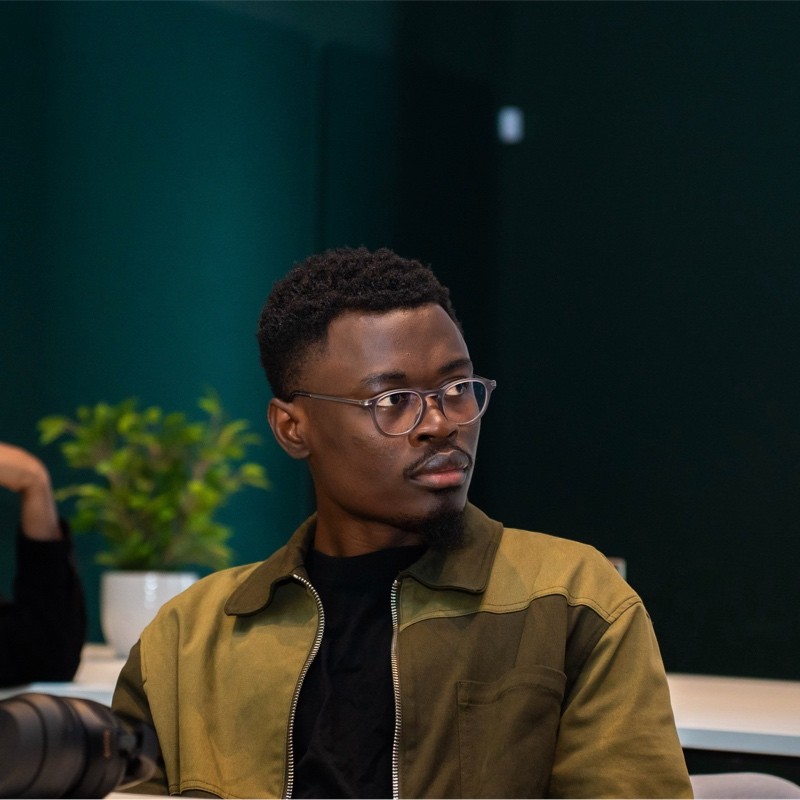
Odohi David
CTO of Grovepay