The End-to-End API & Web Services Management Toolkit
APItoolkit uses AI to help engineering teams observe, manage & test their backend systems and any APIs they depend on.
✓ Privacy controls
✓ GDPR compliant
✓ Security first
Trusted by 3000+ Developers at companies like
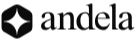


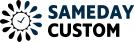
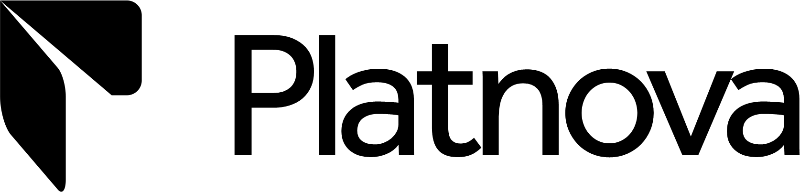
Monitoring & Observability
Monitor Critical Systems and third parties
Gain data-driven insights into your APIs and APIs you depend on. Query user behavior and see all requests made by users or requests you made to third parties.
“APIToolkit allowed us make a drop in rewrite of our PHP service in Golang. Fixing all bugs without customers noticing any changes.”
Michael Okoh CEO @ Thepeer
“We had a major production incident, and the Engineering team didnt need to get involved, because the support team could see via APItoolkit, that the issues were caused by our third party integration, and they could reach out to the team to fix the issue.”
Joshua Chinemezu CEO @ Platnova
E2E API Testing & Synthetic Monitors
Run active e2e monitors on any APIs, and assert if key usecases work as expected.
Our AI even generates these tests and monitors for you, from your OpenAPI/Swagger spec, or from API request logs. Schedule them to run at intervals and configure specific checks and assertions.
“We had to del with very unreliable integration partners, and APItoolkit helped us catch breaking changes they introduced, and armed us better to request better reliability guarantees.”
David Odohi Engineering Lead @ Grovepay
Documentation & API Specification
The best Documentation is one you didnt have to write. Powered by AI and your API usage logs.
Automatically generate API specifications from the live API payloads that are analyzed. Enrich this specification with more context, descriptions, etc., and generate public-facing API documentation or OpenAPI/Swagger specifications.
“We had a lot of issues coming from Merchants of our service, and needed something to track the incoming requests made by these merchants. APItoolkit was exactly what we needed, and even more.”
Michael Akinwonmi CEO @ Payfonte
Scale with security
Built for security and privacy. Use our managed service or host on your own infra.


And so much more...
Stay on top of your APIs, with real-time monitoring, specification, and quality assurance.
Powerful Reports
Get Daily and weekly Reports about the numbers that matter.
Up to date API Docs & Spec
AI generated spec based off your traffic. Approve new changes.
Automatic Changelogs
Maintain a changelog of your APis and APIs your depend on.
Error Analytics
Get deeper insights about errors and the affected users.
Self Host
Don’t let your data leave your server via self hosting.
Alerts & Integrations
Get notified about realtime issues on your terms.
Build with Community
Join us on a mission to build a world from the frustrations of broken API contracts and unreliability.
Loved by Builders
APItoolkit is the intelligent API management system for teams who value peace of mind.
### Frequestly asked
Questions
Some questions others have asked View all FAQ
Express js
Quickly integrate APIToolkit into your express js application
Installation
npm install apitoolkit-express
Integrate
import express from 'express';
import { APIToolkit } from 'apitoolkit-express';
const app = express();
const apitoolkitClient = APIToolkit.NewClient({ apiKey: '' });
app.use(apitoolkitClient.expressMiddleware)
// All controllers should live here
app.get("/", (req, res) => {
});
// ...
// The error handler must be before any other error middleware
// and after all controllers
app.use(apitoolkitClient.errorHandler)
Elixir Phoenix
Quickly integrate APIToolkit into your elixir application
Installation (add to deps)
{:apitoolkit_phoenix, "~> 0.1.1"}
Integrate
// route.ex file
defmodule HelloWeb.Router do
import ApitoolkitPhoenix
pipeline :api do
plug ApitoolkitPhoenix,
config: %{
api_key: "",
}
end
// Automatic error handling
@impl Plug.ErrorHandler
def handle_errors(conn, err) do
conn = report_error(conn, err)
json(conn, %{message: "Something went wrong"})
end
end
Laravel PHP
Quickly integrate APIToolkit into your laravel application
Installation (add to deps)
composer require apitoolkit/apitoolkit-laravel
Set the APITOOLKIT_KEY environment variable to your API key in your .env file.
APITOOLKIT_KEY=xxxxxx-xxxxx-xxxxxx-xxxxxx-xxxxxx
Integrate
[
\APIToolkit\Http\Middleware\APIToolkit::class,
],
];
}
.NET
Quickly integrate APIToolkit into your .NET application
Installation
dotnet add package ApiToolkit.Net
Integrate
var config = new Config
{
Debug = true, # Set debug flags to false in production
ApiKey = "{Your_APIKey}"
};
var client = await APIToolkit.NewClientAsync(config);
# Register the middleware to use the initialized client
app.Use(async (context, next) =>
{
var apiToolkit = new APIToolkit(next, client);
await apiToolkit.InvokeAsync(context);
});
Django
Quickly integrate APIToolkit into your Django application
Installation
pip install apitoolkit-django
Add APIKey to settings.py
APITOOLKIT_KEY = "< YOUR_API_KEY >"
Integrate
MIDDLEWARE = [
...,
'apitoolkit_django.APIToolkit',
...,
]
var config = new Config
{
Debug = true, # Set debug flags to false in production
ApiKey = "{Your_APIKey}"
};
FastAPI
Quickly integrate APIToolkit into your fastapi application
Installation
pip install apitoolkit-fastapi
Integrate
from fastapi import FastAPI
from apitoolkit_fastapi import APIToolkit
app = FastAPI()
apitoolkit = APIToolkit(api_key='')
app.middleware('http')(apitoolkit.middleware)
Flask
Quickly integrate APIToolkit into your flask application
Installation
pip install apitoolkit-flask
Integrate
from flask import Flask
from apitoolkit_flask import APIToolkit
app = Flask(**name**)
apitoolkit = APIToolkit(api_key="", debug=True)
@app.before_request
def before_request():
apitoolkit.beforeRequest()
@app.after_request
def after_request(response):
apitoolkit.afterRequest(response)
return response
Golang Gin
Quickly integrate APIToolkit into your gin application
Installation
go get github.com/apitoolkit/apitoolkit-go
Integrate
package main
import (
"context"
apitoolkit "github.com/apitoolkit/apitoolkit-go"
"github.com/gin-gonic/gin"
)
func main() {
// Initialize the client using your apitoolkit.io generated apikey
apitoolkitClient, err := apitoolkit.NewClient(context.Background(), apitoolkit.Config{APIKey: "YOUR GENERATED API KEY"})
if err != nil {
panic(err)
}
router := gin.New()
// Register with the corresponding middleware of your choice. For Gin router, we use the GinMiddleware method.
router.Use(apitoolkitClient.GinMiddleware)
}
Adonis JS
Quickly integrate APIToolkit into your adonis js application
Installation
npm install apitoolkit-adonis
Configure the package
node ace configure apitoolkit-adonis
Set APIKEY in /conf/apitoolkit
export const apitoolkitConfig = {
apiKey: "",
};
Integrate
Server.middleware.register([
() => import("@ioc:Adonis/Core/BodyParser"),
() => import("@ioc:APIToolkit"),
]);
Fastify JS
Quickly integrate APIToolkit into your Fastify js application
Installation
npm install apitoolkit-fastify
Integrate
import APIToolkit from 'apitoolkit-fastify';
import Fastify from 'fastify';
const fastify = Fastify();
// Create and initialize an instance of the APIToolkit
const apittoolkitClient = APIToolkit.NewClient({
apiKey: 'YOUR_API_KEY',
fastify,
});
apitoolkitClient.init();
Nest JS
Quickly integrate APIToolkit into your nest js application
Installation
npm install apitoolkit-express
Integrate
import { NestFactory } from '@nestjs/core';
import { APIToolkit } from 'apitoolkit-express';
import { AppModule } from './app.module';
//
async function bootstrap() {
const apiToolkitClient = APIToolkit.NewClient({
apikey: '',
});
const app = await NestFactory.create(AppModule);
app.use(apiToolkitClient.expressMiddleware);
await app.listen(3000);
}
//
bootstrap();
Golang Gorilla Mux
Quickly integrate APIToolkit into your Golang Gorilla Mux application
Installation
go get -u github.com/gorilla/mux
Integrate
package main
import (
"context"
"net/http"
"github.com/gorilla/mux"
apitoolkit "github.com/apitoolkit/apitoolkit-go"
)
func main() {
ctx := context.Background()
// Initialize the client using your generated apikey
apitoolkitClient, err := apitoolkit.NewClient(ctx, apitoolkit.Config{APIKey: ""})
if err != nil {
panic(err)
}
r := mux.NewRouter()
// Register middleware
r.Use(apitoolkitClient.GorillaMuxMiddleware)
}
Symfony PHP
Quickly integrate APIToolkit into your Symfony PHP application
Installation
composer require apitoolkit/apitoolkit-symfony
Set the APITOOLKIT_KEY environment variable to your API key in your .env file.
APITOOLKIT_KEY=xxxxxx-xxxxx-xxxxxx-xxxxxx-xxxxxx
Integrate (services.yaml)
services:
APIToolkit\EventSubscriber\APIToolkitService:
arguments:
$apiKey: '%env(APITOOLKIT_KEY)%'
# Optional: if you want to cache login result add this cache poll instance via setter injection
calls:
- setCachePool: ['@PutYourCachePoolServiceHere']
tags:
- { name: 'kernel.event_subscriber' }
Golang Native
Quickly integrate APIToolkit into your native Golang application
Installation
go get -u github.com/apitoolkit/apitoolkit-go
Integrate
package main
import (
"net/http"
"context"
apitoolkit "github.com/apitoolkit/apitoolkit-go"
)
func main() {
// Initialize APIToolkit client with your generated API key
ctx := context.Background()
apitoolkitClient, err := apitoolkit.NewClient(ctx, apitoolkit.Config{APIKey: "YOUR_GENERATED_API_KEY"})
if err != nil {
panic(err)
}
http.Handle("/", apitoolkitClient.Middleware(http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) {
w.Write([]byte("Hello, World!"))
})))
http.ListenAndServe(":8080", nil)
}
Integrate APIToolkit
We support 14+ web frameworks (if we don't support your framework, email [email protected] and we'll create an SDK for you ASAP)